Pandas的Groupby
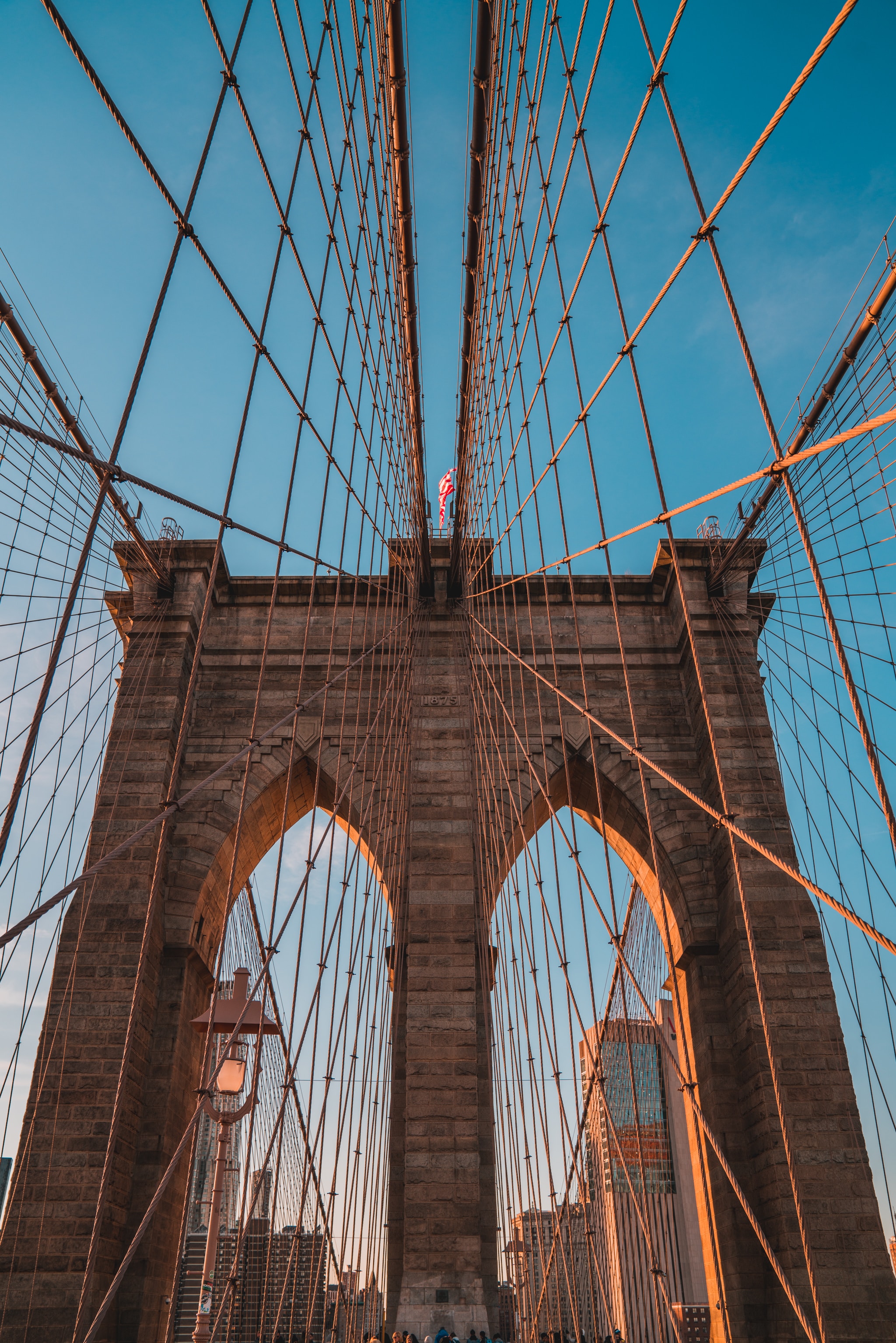
前言
一般需要对数据做分割/处理/合并的时候会使用groupby
, groupby
的意思类似sql语句的分组. 对一个DataFrame
做分割、处理、合并的过程一般如下图所示, 通过这样的流程能做到聚合数据的能力。
分割
官方叫split
, 是把数据依照某种条件分组. 对一个DataFrame使用groupby就达到了split
.
1 | In [1]: import pandas as pd |
处理
apply
对每个分割好的group运行某个(多个)函数. 在这一步我们可能会使用到如下方法:
aggregation
的作用是统计组里的数据: 如平均值, 求和, 计数. 从下面的例子可以看出来,aggregation
后得到一个以groupby
参数作为groupname
,
并且以它为index
的DataFrame
; 如果是通过多个值groupby
的那么结果是以MultiIndex
为索引, 当然index是可以通过as_index
来设置的(.reset_index()
也可以得到相同效果).aggregation
函数会排除NA
值.
1 | In [6]: group_a.agg(sum) ## 注意, 这里如果传递[sum]将会对所有column做sum |
所有的Aggregation
函数如下表, 它们返回的对象一般会减少维度:
agg()
是可以一次运作多个函数的, 注意下面这个函数是对于grouped Series
, 即切出了一个series
操作的, 返回的是dataframe
.
1 | In [30]: group_a['C'].agg([sum, min]) |
如果对一个groupby
Dataframe
应用多个函数, 那么每个column都会有各自的聚合函数列:
1 | In [63]: df.groupby('A').agg([sum, min,max]) |
agg的函数也可以通过lambda
把所有列改成标量值:
1 | In [17]: df.groudby('A').agg(lambda x:1) |
通过给agg()
传递dict
可以对Dataframe的不同column应用不同的聚合. 这样得出的column没有顺序的, 想要有顺序需要使用OrderedDict
1 | In [51]: df.groupby('A').agg({'B': sum, 'C': min}) |
transformation
transform
方法把一个groupby对象做转变, 返回一个和grouped对象一样index
的对象(这点和agg
不同,agg
通常返回维度减少的数据). 这个transform
实际传入的函数必须满足:- 返回和group chunk一样大小的对象, 或者返回的对象大小可以
broadcastable
到group chunk size(broadcasting
是numpy的术语, 描述了numpy对待不同形状阵列时如何计算.) - 在group chunk上做一列一列操作. 实际使用的是chunk.apply
- 不能直接(inplace)在group chunk上修改, group chunk需要认为是不可改变的,
grouped.transform(lambda x: x.fillna(inplace=True))
这样的直接修改可能带来意外结果. - 也可以直接对整个chunk做操作
- 返回和group chunk一样大小的对象, 或者返回的对象大小可以
这里有个例子,讲述了如何使用transform
减少处理的逻辑http://pbpython.com/pandas_transform.html
filteration
使用filter
函数可以返回原来对象的一个子集.filter
的参数是个应用到整个组的函数, 其返回为True/False.
1 | In [115]: sf = pd.Series([1, 1, 2, 3, 3, 3]) |
合并
combining
是再把处理好的数据组合一起.
- Post title:Pandas的Groupby
- Post author:Kopei
- Create time:2018-08-31 00:00:00
- Post link:https://kopei.github.io/2018/08/30/python-2018-08-31-pandas/
- Copyright Notice:All articles in this blog are licensed under BY-NC-SA unless stating additionally.
Comments